What they're saying
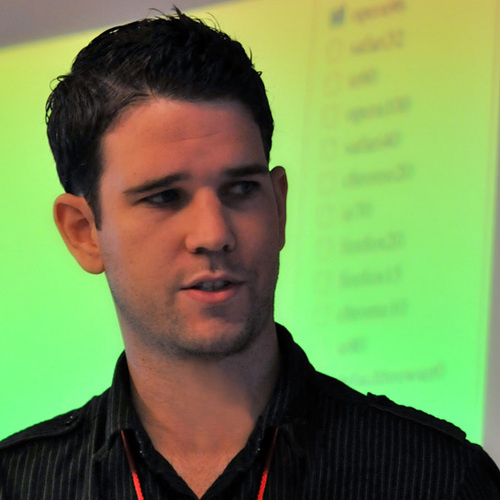
I've been recommending MEjs to my audiences in my html5 talks recently. great stuffPaul Irish
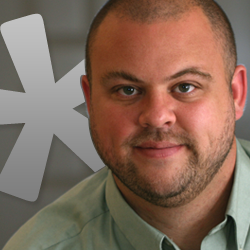
Now that we have this, it's a done deal!Chris Coyier
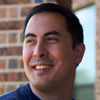
John and I have kids about the same age, and we both like JavaScript.Nathan Smith
How It Works
Flash Fallbackforward
Instead of offering an HTML5 player to modern browsers and a totally separate Flash player to older browsers, MediaElement.js upgrades them with custom Flash and Silverlight plugins that mimic the HTML5 MediaElement API.
Magic happens, and then IE6-8 supports <video>
and <audio>
,
Firefox and Opera support h.264, and Safari and IE9 support WebM
(*if Adobe makes good on promises to support VP8 in Flash).
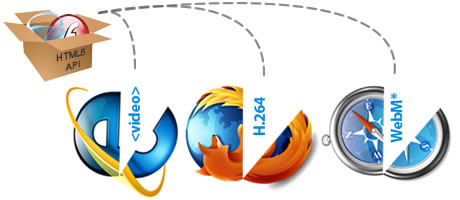
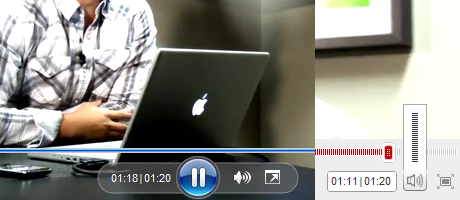
Consistent HTML/CSS Player
Once the browsers have been upgraded so that they all “support” HTML5,
MediaElement builds a fully skinnable player with features like support for the
<track>
element, fullscreen video, and even Ambilight.
Your visitors get a consistent experience regardless of what codecs and plugins their browser supports. And you become a mad HTML5 genius.
Examples & Plugins
You can control what buttons appear on the control bar by setting the {features:['playpause','backlight']}
array (see below).
You can also add your own plugins such as the Backlight example below.
Installation
Setup MIME-types
On Linux/Apache servers, create a filed called .htaccess
with the following text and upload it to the root of your website
AddType video/ogg .ogv
AddType video/mp4 .mp4
AddType video/webm .webm
On Windows/IIS servers, pleaes follow Microsoft's instructions on how to add/edit MIME types on IIS6 and IIS7.
Setup for Local Files (and Flash)
If you are working with local files and plan to test Flash playback, make sure you go to the Flash Security Settings page and add your working directory. Also, things tend to work best when you use absolute paths.
Add Scripts and Stylesheet
Download the latest source code from http://github.com/johndyer/mediaelement/
<script src="jquery.js"></script>
<script src="mediaelement-and-player.min.js"></script>
<link rel="stylesheet" href="mediaelementplayer.css" />
Note: to support IE6-8, this code must appear in the <head> tag. If you cannot place the MediaElement.js code in the <head> you need to install something like html5shiv.
Option A: Single h.264 file
If your users have JavaScript and Flash, this is the easist route for all browsers and mobile devices (the drawback is that h.264 is not fully open and only works in IE9 and Safari under HTML5.)
<video src="myvideo.mp4" width="320" height="240"></video>
Option B: Multiple codecs with Flash fallback
This includes multiple codecs for various browsers (h.264 for IE9, Safari, and mobile browsers; WebM for Firefox 4, Chrome, and Opera; Ogg for Firefox 3) as well as a Flash fallback for non HTML5 browsers with JavaScript disabled. Originally conceived by Kroc Camen as "Video for Everybody."
<video width="320" height="240" poster="poster.jpg" controls="controls" preload="none">
<!-- MP4 for Safari, IE9, iPhone, iPad, Android, and Windows Phone 7 -->
<source type="video/mp4" src="myvideo.mp4" />
<!-- WebM/VP8 for Firefox4, Opera, and Chrome -->
<source type="video/webm" src="myvideo.webm" />
<!-- Ogg/Vorbis for older Firefox and Opera versions -->
<source type="video/ogg" src="myvideo.ogv" />
<!-- Optional: Add subtitles for each language -->
<track kind="subtitles" src="subtitles.srt" srclang="en" />
<!-- Optional: Add chapters -->
<track kind="chapters" src="chapters.srt" srclang="en" />
<!-- Flash fallback for non-HTML5 browsers without JavaScript -->
<object width="320" height="240" type="application/x-shockwave-flash" data="flashmediaelement.swf">
<param name="movie" value="flashmediaelement.swf" />
<param name="flashvars" value="controls=true&file=myvideo.mp4" />
<!-- Image as a last resort -->
<img src="myvideo.jpg" width="320" height="240" title="No video playback capabilities" />
</object>
</video>
Start Player
Convert all <video>
and <audio>
tags to MediaElement.js using jQuery
<script>
// using jQuery
$('video,audio').mediaelementplayer(/* Options */);
</script>
Or explicity create a MediaElementPlayer object for your own use
<script>
// JavaScript object for later use
var player = new MediaElementPlayer('#player',/* Options */);
// ... more code ...
player.pause();
player.setSrc('mynewfile.mp4');
player.play();
</script>
Player Options
$('video').mediaelementplayer({
// if the <video width> is not specified, this is the default
defaultVideoWidth: 480,
// if the <video height> is not specified, this is the default
defaultVideoHeight: 270,
// if set, overrides <video width>
videoWidth: -1,
// if set, overrides <video height>
videoHeight: -1,
// width of audio player
audioWidth: 400,
// height of audio player
audioHeight: 30,
// initial volume when the player starts
startVolume: 0.8,
// useful for <audio> player loops
loop: false,
// enables Flash and Silverlight to resize to content size
enableAutosize: true,
// the order of controls you want on the control bar (and other plugins below)
features: ['playpause','progress','current','duration','tracks','volume','fullscreen'],
// Hide controls when playing and mouse is not over the video
alwaysShowControls: false,
// force iPad's native controls
iPadUseNativeControls: false,
// force iPhone's native controls
iPhoneUseNativeControls: false,
// force Android's native controls
AndroidUseNativeControls: false,
// forces the hour marker (##:00:00)
alwaysShowHours: false,
// show framecount in timecode (##:00:00:00)
showTimecodeFrameCount: false,
// used when showTimecodeFrameCount is set to true
framesPerSecond: 25,
// turns keyboard support on and off for this instance
enableKeyboard: true,
// when this player starts, it will pause other players
pauseOtherPlayers: true,
// array of keyboard commands
keyActions: []
});
API
HTML5/MediaElement API
MediaElementPlayer is a complete audio and video player, but you can also use just the MediaElement object which replaces <video> and <audio> with a Flash player that mimics the properties, methods, and events of HTML MediaElement API. However, there a few changes, most notably on setter properties.
Properties
HTML5 | MediaElement |
---|---|
paused (get) | paused (get) |
ended (get) | ended (get) |
seeking (get) | seeking (get) |
duration (get) | duration (get) |
playbackRate | n/a |
defaultPlaybackRate | n/a |
seekable | n/a |
played | n/a |
muted (get/set) | muted (get), setMuted() |
volume (get/set) | volume (get), setVolume() |
currentTime (get/set) | currentTime (get), setCurrentTime() |
src(get/set) | src (get), setSrc() |
Methods
HTML5 | MediaElement |
---|---|
play() | play() |
pause() | pause() |
load() | load() |
N/A | stop()* |
(*) Note: HTML5 defines a "pause" method, but not a "stop" method. The "stop" method is only present to support Flash RTMP streaming, so you should use the "pause" method in all other cases.
Events
HTML5 | MediaElement |
---|---|
loadeddata | loadeddata |
progress | progress |
timeupdate | timeupdate |
seeked | seeked |
canplay | canplay |
play | play |
playing | playing |
pause | pause |
loadedmetadata | loadedmetadata |
ended | ended |
volumechange | volumechange |
MediaElement Example
This show how to use the MediaElement object, independently of the MediaElementPlayer and jQuery libraries.
Simple <video>
tag and timer
<video id="player1" src="myvideo.mp4" width="320" height="240"></video>
<br />
Time: <span id="current-time"></span>
JavaScript startup with all options
new MediaElement('player1', {
// shows debug errors on screen
enablePluginDebug: false,
// remove or reorder to change plugin priority
plugins: ['flash','silverlight'],
// specify to force MediaElement to use a particular video or audio type
type: '',
// path to Flash and Silverlight plugins
pluginPath: '/myjsfiles/',
// name of flash file
flashName: 'flashmediaelement.swf',
// name of silverlight file
silverlightName: 'silverlightmediaelement.xap',
// default if the <video width> is not specified
defaultVideoWidth: 480,
// default if the <video height> is not specified
defaultVideoHeight: 270,
// overrides <video width>
pluginWidth: -1,
// overrides <video height>
pluginHeight: -1,
// rate in milliseconds for Flash and Silverlight to fire the timeupdate event
// larger number is less accurate, but less strain on plugin->JavaScript bridge
timerRate: 250,
// method that fires when the Flash or Silverlight object is ready
success: function (mediaElement, domObject) {
// add event listener
mediaElement.addEventListener('timeupdate', function(e) {
document.getElementById('current-time').innerHTML = mediaElement.currentTime;
}, false);
// call the play method
mediaElement.play();
},
// fires when a problem is detected
error: function () {
}
});